ScheduledExecutorService
简单看下其继承关系的类图如下:
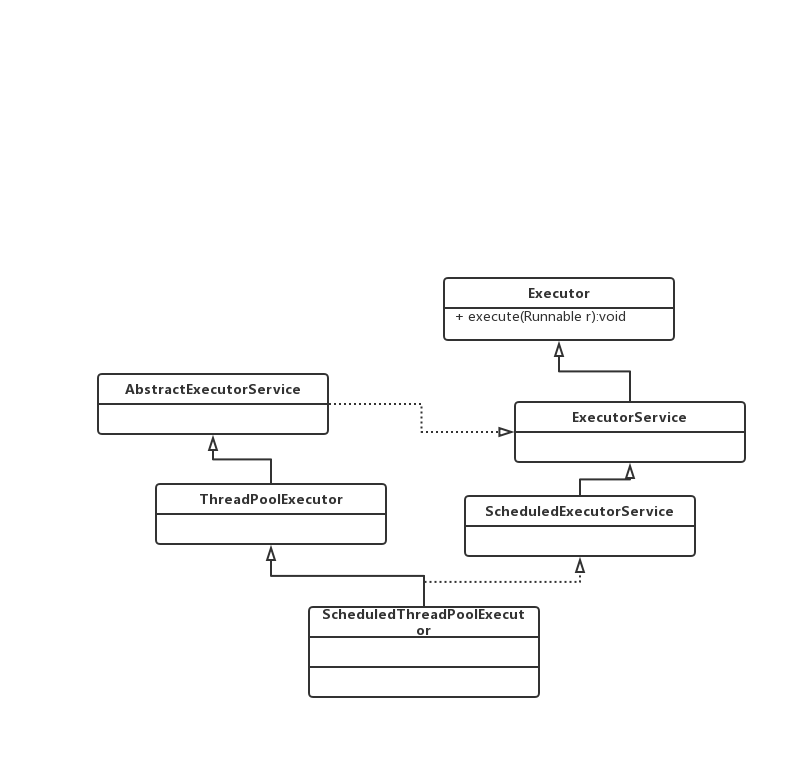
Executor
Executor定义了一个线程池的最核心的操作execute,帮助我们将任务提交和任务具体执行、调度进行解耦。1
void execute(Runnable command);
通过Executor而不是现实的创建线程(new Thread(RunnableTask()).start()
):1
2
3
4Executor executor = anExecutor();
executor.execute(new RunnableTask1());
executor.execute(new RunnableTask2());
...
ExecutorService
ExecutorService提供方法来管理线程池的关闭、提供Future
来追踪一个或多个异步任务的执行进度。ExecutorService可以关闭拒绝新来的任务,其提供了两种关闭方式:
- shutdown() :关闭前允许之前提交的任务先执行完
- shutdownNow():阻止等待的任务并尝试停止正在执行的任务
submit()
方法通过execute()
实现并返回一个Future
,可取消任务的执行或等待任务执行完成。invokeAny
和invokeAll
方法用于批量执行任务,等待至少一个或者全部任务执行完成。1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18void shutdown();
List<Runnable> shutdownNow();
boolean isShutdown();
boolean isTerminated();
boolean awaitTermination(long timeout, TimeUnit unit)
throws InterruptedException;
<T> Future<T> submit(Callable<T> task);
<T> Future<T> submit(Runnable task, T result);
Future<?> submit(Runnable task);
<T> List<Future<T>> invokeAll(Collection<? extends Callable<T>> tasks)
throws InterruptedException;
<T> List<Future<T>> invokeAll(Collection<? extends Callable<T>> tasks, long timeout, TimeUnit unit)
throws InterruptedException;
<T> T invokeAny(Collection<? extends Callable<T>> tasks)
throws InterruptedException, ExecutionException;
<T> T invokeAny(Collection<? extends Callable<T>> tasks,
long timeout, TimeUnit unit)
throws InterruptedException, ExecutionException, TimeoutException;
ScheduledExecutorService
scheduledExecutorService在ExecutorService的基础上加入了定时任务调度的特性,可以调度任务在给定延时执行或在固定周期内执行。schedule()
可创建不同延迟的任务,并返回一个可取消或校验执行的ScheduledFuture<?>
对象。scheduledAtFixedRate()
和scheduledWithFixedDelay()
方法创建周期性任务直达任务取消。
1 | public ScheduledFuture<?> schedule(Runnable command, long delay, TimeUnit unit); |
ScheduledThreadPoolExecutor
ScheduledThreadPoolExecutor是一个可以设置固定延迟或周期性执行任务的ThreadPoolExecutor。相对于Timer来说,当需要多个工作线程,或需要更加灵活 或需要ThreadPoolExecutor相关功能,ScheduledThreadPoolExecutor会更适合。
1 | //ScheduledThreadPoolExecutor |
1 | //ScheduledThreadPoolExecutor$ScheduledFutureTask |
ScheduledThreadPoolExecutor相对于ThreadPoolExecutor,通过将Runnable
任务封装为ScheduledFutureTask
,内部使用的BlockingQueue
为自定义的DelayedWorkQueue
来完成固定延迟或周期性调度任务,ScheduledFutureTask
其类图如下:
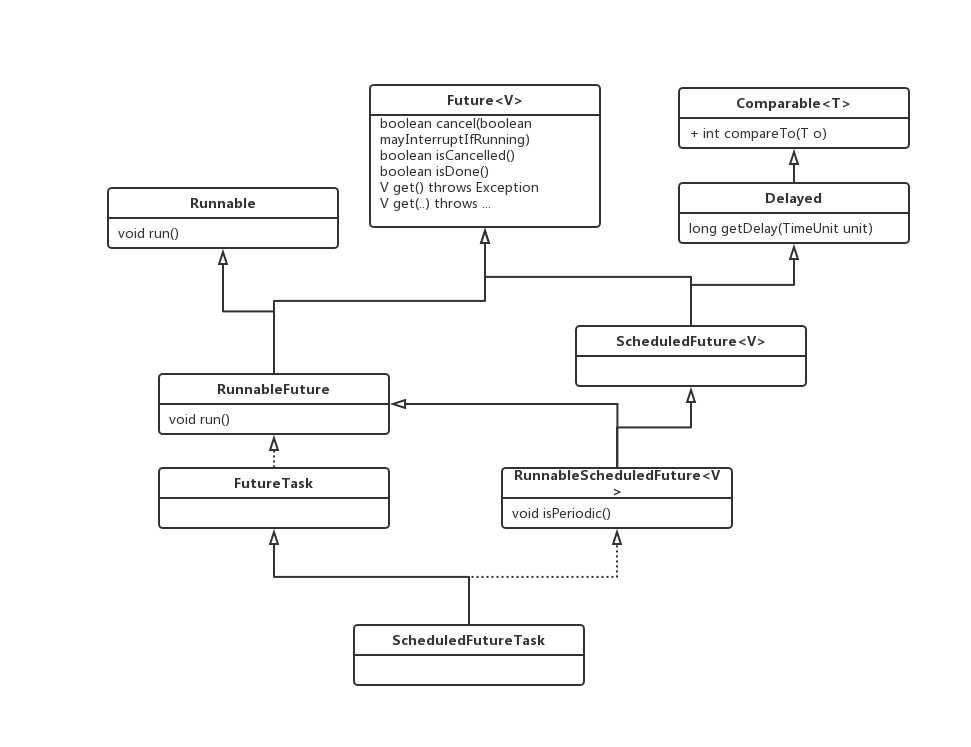
DelayedWorkQueue
其源码类似于PriorityQueue
,核心使用了最小堆来维护数据,加入删除数据时使用siftup
和siftDown
操作来保证最小堆的正确性。ScheduledThreadPoolExecutor
介于此,只需检查最小堆顶部的任务是否达到执行条件即可 故而使用ScheduledThreadPoolExecutor
来执行的定时任务 并不是那么准确