ConcurrentSkipListMap
是基于跳表实现,跳表是一个随机化的数据结构,实质是一种可以进行二分查找的有序链表。跳表在原有的有序链表上面增加了多级索引,通过索引来实现快速查找。
调表不仅能提高搜索性能,同时也能提高插入和删除操作的性能。
其继承关系如下:
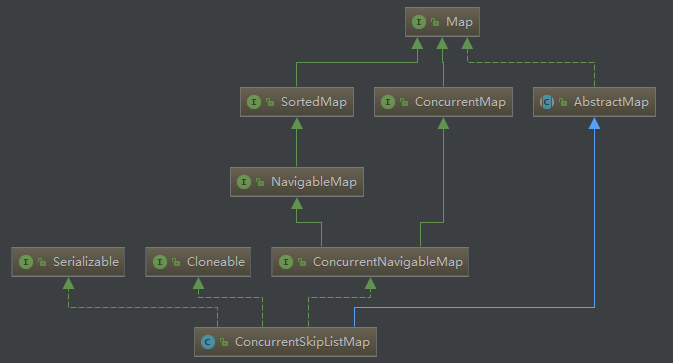
主要属性
1 | public class ConcurrentSkipListMap<K,V> extends AbstractMap<K,V> |
内部类
1 | //数据节点 |
构造器
1 | public ConcurrentSkipListMap() { |
initialize()
1 | private void initialize() { |
添加元素
1 | public V put(K key, V value) { |
删除元素
1 | public V remove(Object key) { |
查找元素
1 | public V get(Object key) { |