WeakHashMap
是一种弱引用map,内部key会存储为弱引用,当JVM GC时 若这些key没有强引用存在时就会被gc回收,下次当我们操作map时会将对应的Entry
这个删除掉,基于这个特性,WeakHashMap
特别适合做缓存。
其继承关系如下:
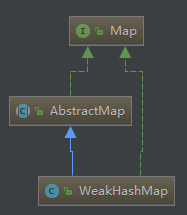
主要属性
1 | public class WeakHashMap<K,V> |
构造器
1 | public WeakHashMap(int initialCapacity, float loadFactor) { |
内部类
1 | private static class Entry<K,V> extends WeakReference<Object> implements Map.Entry<K,V> { |
put(K key,V value)
1 | public V put(K key, V value) { |
resize(int newCapacity)
1 | void resize(int newCapacity) { |
get(Object key)
1 | public V get(Object key) { |
remove(Object key)
1 | public V remove(Object key) { |
expungeStaleEntries()
删除失效的Entry1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29private void expungeStaleEntries() {
//当key失效时gc会自动将对应的Entry添加到引用队列queue中
for (Object x; (x = queue.poll()) != null; ) {
synchronized (queue) {
"unchecked") (
Entry<K,V> e = (Entry<K,V>) x;
int i = indexFor(e.hash, table.length);
Entry<K,V> prev = table[i];
Entry<K,V> p = prev;
while (p != null) {
Entry<K,V> next = p.next;
if (p == e) {
if (prev == e)
table[i] = next;
else
prev.next = next;
// Must not null out e.next;
// stale entries may be in use by a HashIterator
e.value = null; // Help GC
size--;
break;
}
prev = p;
p = next;
}
}
}
}